Go語言寫Web應(yīng)用程序
介紹
涵蓋內(nèi)容:
◆ 為載入和保存方法創(chuàng)建一個(gè)數(shù)據(jù)結(jié)構(gòu)體
◆ 引用http包來創(chuàng)建一個(gè)web應(yīng)用
◆ 引用template包來處理HTML模板
◆ 引用regexp包來驗(yàn)證用戶的輸入
◆ 引用 閉包操作
可能涉及到的知識(shí):
◆ 設(shè)計(jì)經(jīng)驗(yàn)
◆ 明白基礎(chǔ)的web技術(shù)(HTTP,HTML)
◆ 一些UNIX命令行知識(shí)
從這里開始
你要有一個(gè)可以運(yùn)行Go語言的計(jì)算機(jī)或虛擬機(jī),怎么樣安裝Go,請(qǐng)參考安裝Go教程。首先創(chuàng)建一個(gè)目錄,在目錄下創(chuàng)建一個(gè)wiki.go文件,用你喜歡的編輯器打開并輸入以下內(nèi)容:
- package main
- import (
- "fmt"
- "io/ioutil"
- "os"
- )
這fmt,ioutil和os都是go語言的標(biāo)準(zhǔn)庫,一會(huì)我將增加其他方法和更多的包。
數(shù)據(jù)結(jié)構(gòu)
讓我們聲明一個(gè)數(shù)據(jù)結(jié)構(gòu),這個(gè)結(jié)構(gòu)主要包含兩個(gè)字段,一個(gè)是標(biāo)題,一個(gè)是內(nèi)容。
- type Page struct {
- Title string
- Body []byte
- }
接下來,我們給Page 這個(gè)結(jié)構(gòu)體寫個(gè)保存方法,代碼如下:
- func (p *Page) save() os.Error {
- filename := p.Title + ".txt"
- return ioutil.WriteFile(filename, p.Body, 0600)
- }
這個(gè)方法的簽名是:接收一個(gè)Page結(jié)構(gòu)體指針,返回一個(gè)os.Error錯(cuò)誤。
在一下的代碼中還是用了http包和模板包,具體內(nèi)容參考具體代碼,再這里就不詳細(xì)貼出來了。下面是模板內(nèi)容,把他們放到wiki.go同一目錄下。
編輯頁面 模板e(cuò)idt.html
- <h1>Editing {{.Title |html}}</h1>
- <form action="/save/{{.Title |html}}" method="POST">
- <div><textarea name="body" rows="20" cols="80">{{printf "%s" .Body |html}}</textarea></div>
- <div><input type="submit" value="Save"></div>
- </form>
查看頁面模板view.html
- <h1>{{.Title |html}}</h1>
- <p>[<a href="/edit/{{.Title |html}}">edit</a>]</p>
- <div>{{printf "%s" .Body |html}}</div>
完整代碼:wiki.go
- package main
- import (
- "http"
- "io/ioutil"
- "os"
- "regexp"
- "template"
- )
- type Page struct {
- Title string
- Body []byte
- }
- func (p *Page) save() os.Error {
- filename := p.Title + ".txt"
- return ioutil.WriteFile(filename, p.Body, 0600)
- }
- func loadPage(title string) (*Page, os.Error) {
- filename := title + ".txt"
- body, err := ioutil.ReadFile(filename)
- if err != nil {
- return nil, err
- }
- return &Page{Title: title, Body: body}, nil
- }
- func viewHandler(w http.ResponseWriter, r *http.Request, title string) {
- p, err := loadPage(title)
- if err != nil {
- http.Redirect(w, r, "/edit/"+title, http.StatusFound)
- return
- }
- renderTemplate(w, "view", p)
- }
- func editHandler(w http.ResponseWriter, r *http.Request, title string) {
- p, err := loadPage(title)
- if err != nil {
- p = &Page{Title: title}
- }
- renderTemplate(w, "edit", p)
- }
- func saveHandler(w http.ResponseWriter, r *http.Request, title string) {
- body := r.FormValue("body")
- p := &Page{Title: title, Body: []byte(body)}
- err := p.save()
- if err != nil {
- http.Error(w, err.String(), http.StatusInternalServerError)
- return
- }
- http.Redirect(w, r, "/view/"+title, http.StatusFound)
- }
- var templates = make(map[string]*template.Template)
- func init() {
- for _, tmpl := range []string{"edit", "view"} {
- t := template.Must(template.ParseFile(tmpl + ".html"))
- templates[tmpl] = t
- }
- }
- func renderTemplate(w http.ResponseWriter, tmpl string, p *Page) {
- err := templates[tmpl].Execute(w, p)
- if err != nil {
- http.Error(w, err.String(), http.StatusInternalServerError)
- }
- }
- const lenlenPath = len("/view/")
- var titleValidator = regexp.MustCompile("^[a-zA-Z0-9]+$")
- func makeHandler(fn func(http.ResponseWriter, *http.Request, string)) http.HandlerFunc {
- return func(w http.ResponseWriter, r *http.Request) {
- title := r.URL.Path[lenPath:]
- if !titleValidator.MatchString(title) {
- http.NotFound(w, r)
- return
- }
- fn(w, r, title)
- }
- }
- func main() {
- http.HandleFunc("/view/", makeHandler(viewHandler))
- http.HandleFunc("/edit/", makeHandler(editHandler))
- http.HandleFunc("/save/", makeHandler(saveHandler))
- http.ListenAndServe(":8080", nil)
- }
運(yùn)行測試:
$ 8g wiki.go
$ 8l wiki.8
$ ./8.out
在地址欄輸入地址:http://localhost:8080/view/aNewPage
效果圖:
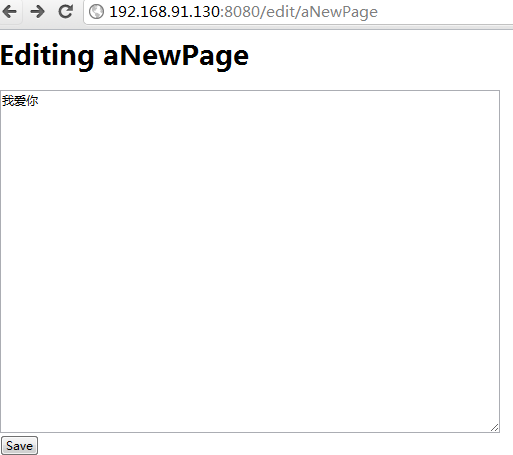
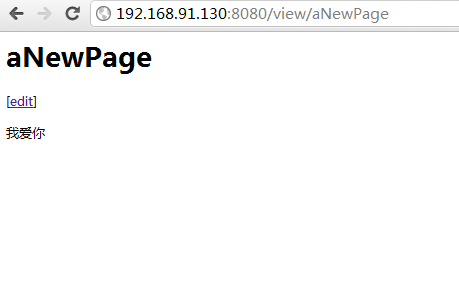
原文:http://www.cnblogs.com/zitsing/archive/2012/03/19/2406226.html
【編輯推薦】
- Go語言源碼可追溯到1972 年?
- 谷歌發(fā)布Go編程語言***候選版
- 用Google Go語言實(shí)現(xiàn)http共享
- Google Web App開發(fā)指南之構(gòu)建優(yōu)秀的Web Apps
- Google Web App開發(fā)指南:交互設(shè)計(jì)