JavaScript包含各種對典型編程思想有用的一些技巧,在實際開發(fā)中,我們通常希望減少代碼行數(shù);因此,今天這些技巧代碼希望可以幫助到你。
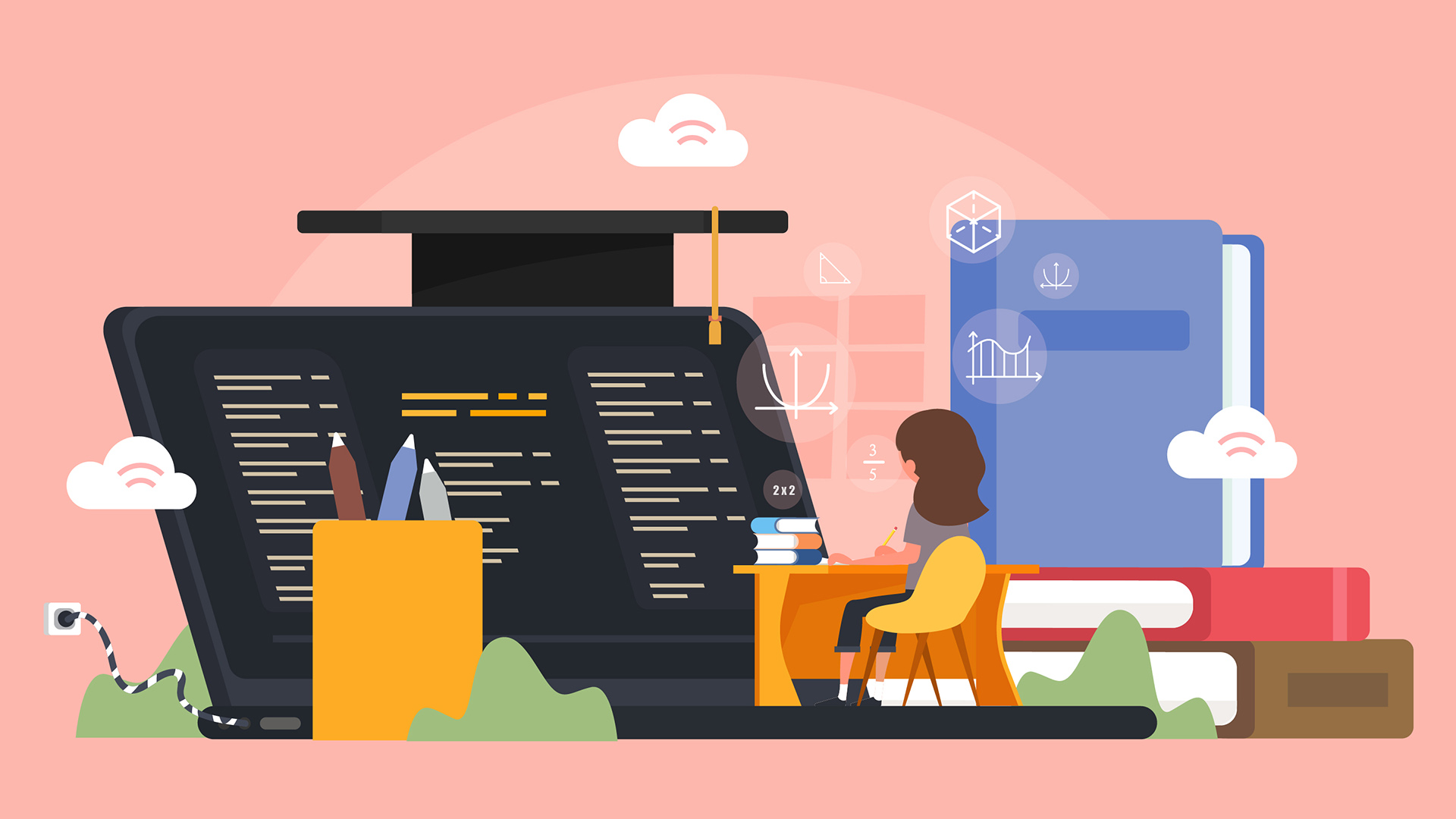
現(xiàn)在我們就開始今天的內(nèi)容吧。
1、與Javascript對象相關(guān)的
01)、對象解構(gòu)
將對象的值解構(gòu)為變量是另一種在傳統(tǒng)點表示法之外讀取其值的方法。
下面的示例比較了用于讀取對象值的經(jīng)典點表示法和對象解構(gòu)快捷方式。
const restaurant = {
name: "Classico Italiano",
menu: ["Garlic", "Bread", "Salad", "Pizza", "Pasta"],
openingHours: {
friday: { open: 11, close: 24 },
saturday: { open: 12, close: 23 },
},
};
// Longhand
console.log("value of friday in restaurant:", restaurant.openingHours.friday);
console.log("value of name in restaurant:", restaurant.name);
// Shorthand
const { name, openingHours } = restaurant;
const { openingHours: { friday } } = restaurant;
//we can go further and get the value of open in friday
const { openingHours: { friday: { open } } } = restaurant;
console.log(name, friday, open);
02)、Object.entries()
Object.entries() 是 ES8 中引入的一項功能,它允許您將文字對象轉(zhuǎn)換為鍵/值對數(shù)組。
const credits = {
producer: "Open Replay",
editor: "Federico",
assistant: "John",
};
const arr = Object.entries(credits);
console.log(arr);
Output: [
["producer", "Open Replay"],
["editor", "Federico"],
["assistant", "John"],
];
03)、Object.values()
Object.values() 也是 ES8 中引入的新特性,與 Object 具有類似的功能。entries() 但這沒有關(guān)鍵部分:
const credits = {
producer: "Open Replay",
editor: "Federico",
assistant: "John",
};
const arr = Object.values(credits);
console.log(arr);
Output: ["Open Replay", "Federico", "John"];
04)、對象循環(huán)速記
傳統(tǒng)的 JavaScript for 循環(huán)語法是 for (let i = 0; i < x; i++) { … }。通過引用迭代器的數(shù)組長度,此循環(huán)語法可用于遍歷數(shù)組。共有三個 for 循環(huán)快捷方式,它們提供了用于遍歷數(shù)組中的對象的各種方法:
for…of 創(chuàng)建一個循環(huán)遍歷內(nèi)置字符串、數(shù)組和類似數(shù)組的對象,甚至是 Nodelist
for...in 在記錄屬性名稱和值的字符串時訪問數(shù)組的索引和對象文字上的鍵。
Array.forEach 使用回調(diào)函數(shù)對數(shù)組元素及其索引執(zhí)行操作
const arr = ["Yes", "No", "Maybe"];
// Longhand
for (let i = 0; i < arr.length; i++) {
console.log("Here is item: ", arr[i]);
}
// Shorthand
for (let str of arr) {
console.log("Here is item: ", str);
}
arr.forEach((str) => {
console.log("Here is item: ", str);
});
for (let index in arr) {
console.log(`Item at index ${index} is ${arr[index]}`);
}
// For object literals
const obj = { a: 1, b: 3, c: 5 };
for (let key in obj) {
console.log(`Value at key ${key} is ${obj[key]}`);
}
05)、對象屬性簡寫
在 JavaScript 中定義對象字面量讓生活變得更輕松。ES6 在為對象賦予屬性方面提供了更多的簡單性。如果變量名和對象鍵相同,則可以使用速記符號。
通過在對象字面量中聲明變量,您可以在 JavaScript 中快速將屬性分配給對象。為此,必須使用預(yù)期的鍵命名變量。這通常在您已經(jīng)有一個與對象屬性同名的變量時使用。
const a = 1;
const b = 2;
const c = 3;
// Longhand
const obj = {
a: a,
b: b,
c: c,
};
// Shorthand
const obj = { a, b, c };
06)、Javascript For 循環(huán)
如果你想要簡單的 JavaScript 并且不想依賴第三方庫,這個小技巧很有用。
// Longhand:
const fruits = ['mango', 'peach', 'banana'];
for (let i = 0; i < fruits.length; i++) { /* something */ }
// Shorthand:
for (let fruit of fruits) { /* something */ }
如果您想訪問文字對象中的鍵,這也適用:
const obj = { continent: "Africa", country: "Ghana", city: "Accra" };
for (let key in obj) console.log(key); // output: continent, country, city
07)、Array.forEach 的簡寫:
function logArrayElements(element, index, array) {
console.log("a[" + index + "] = " + element);
}
[2, 4, 6].forEach(logArrayElements);
// a[0] = 2
// a[1] = 4
// a[2] = 6
2、與Javascript數(shù)組相關(guān)的
01)、數(shù)組解構(gòu)
ES6 的一個有趣的新增功能是解構(gòu)賦值。解構(gòu)是一種 JavaScript 表達(dá)式,可以將數(shù)組值或?qū)ο髮傩苑蛛x到單獨的變量中。換句話說,我們可以通過從數(shù)組和對象中提取數(shù)據(jù)來將數(shù)據(jù)分配給變量。
const arr = [2, 3, 4];
// Longhand
const a = arr[0];
const b = arr[1];
const c = arr[2];
// Shorthand
const [a, b, c] = arr;
02)、Spread Operator
得益于 ES6 中引入的展開運算符,JavaScript 代碼使用起來更加高效和愉快。它可以代替特定的數(shù)組函數(shù)。展開運算符中有一系列的三個點,我們可以用它來連接和克隆數(shù)組。
const odd = [3, 5, 7];
const arr = [1, 2, 3, 4];
// Longhand
const nums = [2, 4, 6].concat(odd);
const arr2 = arr.slice();
// Shorthand
const nums = [2, 4, 6, ...odd];
const arr2 = [...arr];
與 concat() 函數(shù)不同,您可以使用擴(kuò)展運算符將一個數(shù)組插入另一個數(shù)組中的任意位置。
const odd = [3, 5, 7];
const nums = [2, ...odd, 4, 6]; // 2 3 5 7 4 6
3、與Javascript字符串相關(guān)
01)、多行字符串
如果您曾經(jīng)發(fā)現(xiàn)自己需要在代碼中編寫多行字符串,那么您可以這樣寫:
// Longhand
const lorem =
"Lorem, ipsum dolor sit amet" +
"consectetur adipisicing elit." +
" Quod eaque sint voluptatem aspernatur provident" +
"facere a dolorem consectetur illo reiciendis autem" +
"culpa eos itaque maxime quis iusto quisquam" +
"deserunt similique, dolores dolor repudiandae!" +
"Eaque, facere? Unde architecto ratione minus eaque" +
"accusamus, accusantium facere, sunt" +
"quia ex dolorem fuga, laboriosam atque.";
但是有一種更簡單的方法,只需使用反引號即可完成。
// Shorthand:
const lorem = `Lorem, ipsum dolor sit amet
consectetur adipisicing elit.
Quod eaque sint voluptatem aspernatur provident
facere a dolorem consectetur illo reiciendis autem
culpa eos itaque maxime quis iusto quisquam
deserunt similique, dolores dolor repudiandae!
Eaque, facere? Unde architecto ratione minus eaque
accusamus, accusantium facere, sunt
quia ex dolorem fuga, laboriosam atque.`;
02)、將字符串轉(zhuǎn)換為數(shù)字
您的代碼可能偶爾會收到必須以數(shù)字格式處理的字符串格式的數(shù)據(jù)。這不是一個大問題;我們可以快速轉(zhuǎn)換它。
// Longhand
const num1 = parseInt('1000');
const num2 = parseFloat('1000.01')
// Shorthand
const num1 = +'1000'; //converts to int datatype
const num2 = +'1000.01'; //converts to float data type
03)、模板文字
我們可以在不使用 (+) 的情況下將許多變量附加到字符串中。模板文字在這里派上用場。用反引號包裹你的字符串,用 ${ } 包裹你的變量,以利用模板文字。
const fullName = "Ama Johnson";
const job = "teacher";
const birthYear = 2000;
const year = 2025;
// Longhand
const Fullstr =
"I am " + fullName + ", a " + (year - birthYear) + " years old " + job + ".";
// Shorthand
const Fullstr = `I am ${fullName}, a ${year - birthYear} years old ${job}. `;
模板字面量為我們省去了許多使用 + 運算符連接字符串的麻煩。
4、與指數(shù)有關(guān)的
01)、指數(shù)冪速記
將第一個操作數(shù)乘以第二個操作數(shù)的冪的結(jié)果是求冪運算符 ** 返回的結(jié)果。它與 Math.pow 相同,只是 BigInts 也被接受為操作數(shù)。
// Longhand
Math.pow(2, 3); //8
Math.pow(2, 2); //4
Math.pow(4, 3); //64
// Shorthand
2 ** 3; //8
2 ** 4; //4
4 ** 3; //64
02)、十進(jìn)制底指數(shù)
這個可能以前見過。本質(zhì)上,這是一種寫整數(shù)的奇特方法,沒有最后的零。例如,表達(dá)式 1e8 表示 1 后跟八個零。它表示十進(jìn)制基數(shù) 100,000,000,JavaScript 將其解釋為浮點類型。
// Longhand
for (let i = 0; i < 10000000; i++) { /* something */ }
// Shorthand
for (let i = 0; i < 1e7; i++) { /* something */ }
// All the below will evaluate to true
1e0 === 1;
1e1 === 10;
1e2 === 100;
1e3 === 1000;
1e4 === 10000;
1e5 === 100000;
1e6 === 1000000;
1e7 === 10000000;
1e8 === 100000000;
5、其他操作的 JavaScript 簡寫?
01)、短路評估
短路評估也可以替代 if...else 子句。當(dāng)變量的預(yù)期值為 false 時,此快捷方式使用邏輯或運算符 || 為變量提供默認(rèn)值。
let str = "";
let finalStr;
// Longhand
if (str !== null && str !== undefined && str != "") {
finalStr = str;
} else {
finalStr = "default string";
}
// Shorthand
let finalStr = str || "default string"; // 'default string'
02)、默認(rèn)參數(shù)
if 語句用于定義函數(shù)參數(shù)的默認(rèn)值。在 ES6 中,您可以在函數(shù)聲明本身中定義默認(rèn)值。如果沒有傳遞任何值或未定義,則默認(rèn)函數(shù)參數(shù)允許使用默認(rèn)值初始化參數(shù)。
以前都是在函數(shù)體中測試參數(shù)值,如果沒有定義就賦值。
默認(rèn)情況下,JavaScript 中的函數(shù)參數(shù)是未定義的。然而,設(shè)置不同的默認(rèn)設(shè)置通常是有利的。在這里,默認(rèn)設(shè)置可能很有用。
// Longhand
function volume(a, b, c) {
if (b === undefined) b = 3;
if (c === undefined) c = 4;
return a * b * c;
}
// Shorthand
function volume(a, b = 3, c = 4) {
return a * b * c;
}
03)、隱式返回函數(shù)
我們經(jīng)常使用關(guān)鍵字 return 來指示函數(shù)的最終輸出。單語句箭頭函數(shù)將隱式返回其計算結(jié)果(該函數(shù)必須省略方括號 {} 才能這樣做)。
對于多行語句,例如表達(dá)式,我們可以將返回表達(dá)式包裹在括號 () 中。
function capitalize(name) {
return name.toUpperCase();
}
function add(numG, numH) {
return numG + numH;
}
// Shorthand
const capitalize = (name) => name.toUpperCase();
const add = (numG, numH) => numG + numH;
// Shorthand TypeScript (specifying variable type)
const capitalize = (name: string) => name.toUpperCase();
const add = (numG: number, numH: number) => numG + numH;
04)、聲明變量
在函數(shù)的開頭聲明變量賦值是個好主意。這種精簡方法可以幫助您在一次創(chuàng)建大量變量時節(jié)省大量時間和空間。
// Longhand
let x;
let y;
let z = 3;
// Shorthand
let x, y, z=3;
但是,請注意,出于易讀性考慮,許多人更喜歡用手寫方式聲明變量。
05)、可選鏈接
我們可以使用點表示法訪問對象的鍵或值。我們可以通過可選鏈接更進(jìn)一步并讀取鍵或值,即使我們不確定它們是否存在或是否已設(shè)置。如果鍵不存在,則可選鏈接的值未定義。
下面是一個可選鏈的例子:
const restaurant = {
details: {
name: "Classico Italiano",
menu: ["Garlic", "Bread", "Salad", "Pizza"],
},
};
// Longhand
console.log(
"Name ",
restaurant.hasOwnProperty("details") &&
restaurant.details.hasOwnProperty("name") &&
restaurant.details.name
);
// Shorthand
console.log("Name:", restaurant.details?.name);
06)、雙位非運算符
JavaScript 中的內(nèi)置 Math 對象通常用于訪問數(shù)學(xué)函數(shù)和常量。一些函數(shù)提供了有用的技術(shù),讓我們可以在不引用 Math 對象的情況下調(diào)用它們。
例如,我們可以通過使用兩次按位非運算符來獲得整數(shù)的 Math.floor() ~~。
const num = 7.5;
// Longhand
const floorNum = Math.floor(num); // 7
// Shorthand
const floorNum = ~~num; // 7
寫在最后
這些是已被證明的一項寶貴技巧,因為它簡潔明了,使編碼變得有趣并且代碼行易于記憶。但是請記住,它們的使用不能以犧牲代碼的其他方面為代價。如果您覺得我今天分享的內(nèi)容有用的話,請點贊我,關(guān)注我,并將它分享給你的朋友,也許能夠幫助到他。